Basics of Object Oriented programming - Part 1
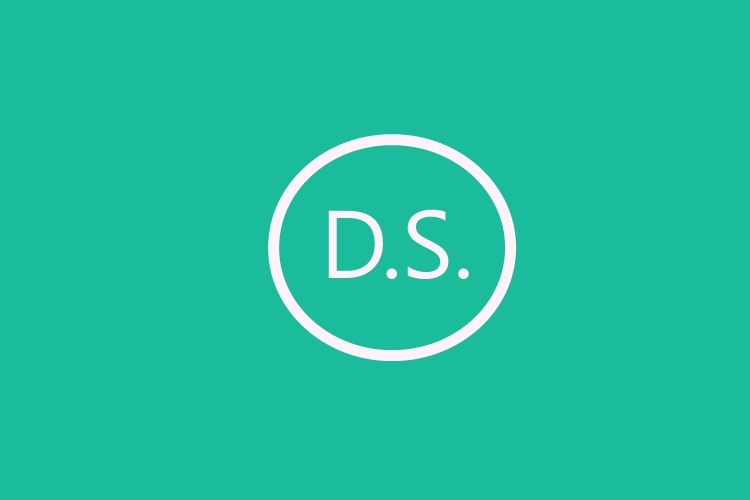
Basics of Object Oriented Programming
Within this introductory tutorial, we are going to introduce you with the basics of object oriented programming. We are going to cover the following topics:
- What does classes consist of?
- What to inherit?
- What is main used for?
- Instance variable vs local variable
- How to decide if a class should extend another class?
- Uses of inheritance
- Other points to keep in mind
- Example related to object oriented programming
What does classes consist of?
A class is like the blueprint of the house, they contain everything necessary to create the house, to define the structure of the program. Within that blueprint, it contains fields/ instance variable and member functions. These two things shape the entire blueprint or shall we say as the class.
Consider another example, suppose we have vehicle, now a vehicle can be a truck, car or bicycle. Here, vehicle is an example of class and various types of vehicles are the subclasses. Now, these classes cannot be accessed without an object.
Hope you have a brief understanding of classes. We would discuss classes and objects later in this tutorial to uderstand better.
What to inherit?
- Look out for things that classes have in common
- Abstract out those features
- Override or extend methods that are useful
Suppose you have an animal class, having function move, eat and getName. Now there are another two classes called Bird which moves and have a member function as move and dog which has a member function as dig hole.
Now, digHole method is not present in animal class, so it would extends the animal subclass using extends keyword. Whereas, the bird subclass contains the method present in the superclass and would override the animal class’s move method.
If a subclass inherits a superclass, then all of the member function and variables are defiend in the subclass.
In the subclass, you define only the changes related to the subclass.
What is main for?
Main are used to create objects that interact with the main class.
Example from github snippets
Instance variable vs local variable
Instance variable are created inside classes and local variable are created inside methods.
How to decide if a class should extend another class?
Use “Has A” vs “Is A” method
Is A helps to decide if a class should extend another class. Example: is Dog an Animal, if answer comes out to yes then it should probably inherit the superclass.
Has A helps decide if something is a field. For example Dog has a height
Don’t inherit to just to reuse code, use the “Is A” principle and check if it should inherit or not. If it doesn’t work, probably inheritence doesn’t work either.
Uses of Inheritence
- To avoid dublicate code
- Changes in the superclass are reflected everywhere in thre subclasses as well.
Other points to keep in mind
- Everything is passed by value in java
- Objects are passed by reference
- Use static for passing objects
Example related to object oriented programming is found here on the github link:
Within the next tutorial, we are going to discuss what is polymorphism, give an example related to polymorphism and abstract classes